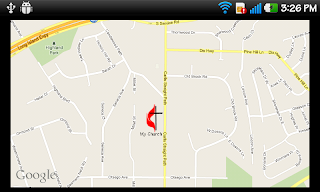
Before you start, you should make sure your project is built by google API, not Android.
package Byung_Yong.sample.mapView; import java.util.List; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Canvas; import android.graphics.Paint; import android.graphics.Point; import android.os.Bundle; import android.util.Log; import com.google.android.maps.GeoPoint; import com.google.android.maps.MapActivity; import com.google.android.maps.MapController; import com.google.android.maps.MapView; import com.google.android.maps.Overlay; import com.google.android.maps.Projection; public class Map extends MapActivity{ MapView mapview; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mapview = (MapView) findViewById(R.id.mapview); mapview.setBuiltInZoomControls(true); mapview.setSatellite(false); GeoPoint gpoint = new GeoPoint(12345678,12345678); MapController controller = mapview.getController(); controller.animateTo(gpoint); controller.setZoom(16); Bitmap image = BitmapFactory.decodeResource(getResources(), R.drawable.place); MyOverlay myoverlay = new MyOverlay(image); ListoverlayList = mapview.getOverlays(); overlayList.add(myoverlay); } @Override protected boolean isRouteDisplayed() { // TODO Auto-generated method stub return false; } } class MyOverlay extends Overlay { Bitmap bitmap; public MyOverlay(Bitmap bitmap){ this.bitmap = bitmap; } @Override public void draw(Canvas canvas, MapView mapView, boolean shadow) { super.draw(canvas, mapView, shadow); Log.d("BB", "overlay"); Paint paint1 = new Paint(); Paint paint2 = new Paint(); paint2.setARGB(255, 0, 0, 0); GeoPoint geopoint = new GeoPoint(12345678,12345678); Point geopix = new Point(); Projection project = mapView.getProjection(); project.toPixels(geopoint, geopix); canvas.drawBitmap(bitmap, geopix.x-50, geopix.y, paint1); canvas.drawText("My Church", geopix.x-50, geopix.y+80, paint2); } }
You can easy to get the location information by searching at Google map.
Press right mouse where you want to mark, and click "What's here".
Then you could see the two 8 digit numbers of coordinate values on search bar or put the mouse on the arrow mark.
Copy the numbers and paste on highlight below.
Manifest will be like this.
Important!!!! Look carefully the highlight part.
You should add "com.google.android.maps" at application and make it "true" just like line 19.
Your Layout can be similar like this.
You should get Google API key by following the direction of below link.
http://mirnauman.wordpress.com/2012/01/26/how-to-get-google-maps-api-key-for-android-issues-and-errors-solved/
No comments:
Post a Comment